
Arrays are one of the obscure concepts in Bash. Its syntax, declaration, accessing, etc all differ from the general view. If properly utilised, arrays are such a powerful concept to use in Bash.
To check the length of the arrays in bash, use the following syntax:
${#array_name[@]}
This is not the only method though. Let's take a look in detail.
Method 1: Use the array length expression
Let's initialise an array like this:
epoch=(1 "Jan" 1970 "Thu")
Print the length of the array by adding a pound #
at the beginning of the array name.
echo ${#array_name[@]}
Syntax to print the number of elements (#) in an array
echo ${#epoch[@]}
Translation: "print (echo) the number of elements (#) in the array 'epoch' (array_name)"
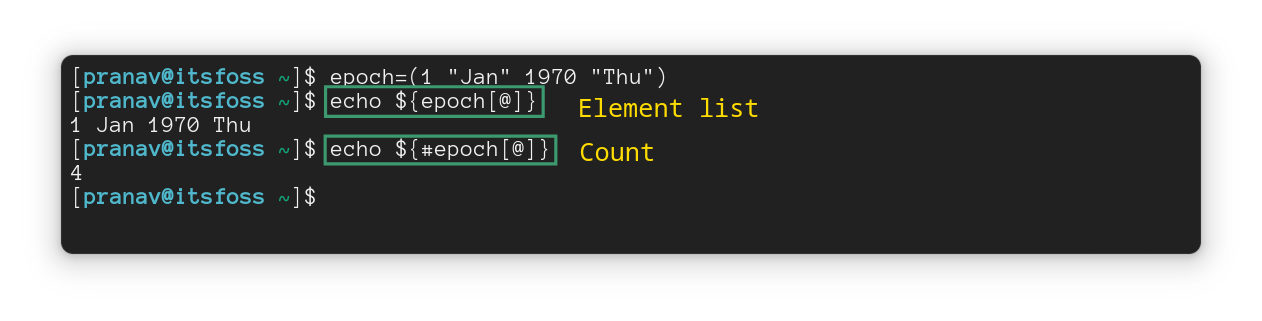
This works and outputs that four elements are present in this array.
A practical example
Where can this notation be used? In a lot of scripts. This method is quick and takes less computational power to check the number of elements in an array.
This format is widely used in loops to terminate the loop after a certain number of steps.
Here's I am creating a script that has a list of applications in programs
array. The script creates another array updated_list
for the programs that are not installed. The final output is the number of programs and their names that will be installed.
#!/bin/bash
# Program list
programs=(grub os-prober networkmanager base-devel linux-headers ntfs-3g mtools dosfstools)
# "pacman -Q": $?=0 success, 1 failure
i=0
updated_list=()
while [[ $i -lt ${#programs[@]} ]]; do
program=${programs[$i]}
# Search for installed package (and hide errors from output)
pacman -Q $program 2> /dev/null #check for package
# (if exit code != 0, then add it to list)
if [[ $? -ne 0 ]]; then updated_list+=($program); fi
((i++))
done
echo "${#updated_list[@]} programs to install: ${updated_list[@]}"
Let's break down this little script:
programs
: This is the array that contains the list of programs to be installed.i=0
: initialisation of the variable$i
for the loop, which increments at the end of the loop body via((i++))
[mathematical expressions must be bounded by double parentheses].while []; do ..... done
: This is the while loop. Its purpose is to remove the programs that are already installed in the system, hence minimising the elements in the list.- The
pacman -Q <package_name>
: Pacman is the package manager of Arch Linux. The-Q
tag stands for query, which is used to search for installed packages in the local system. It returns the exit code 0 upon success, and 1 upon failure (in finding the package).
There's the ${#programs[@]}
expression, which means the number of elements in the array 'program'. From the declaration, its value is '8'. It prevents the loop from executing forever.
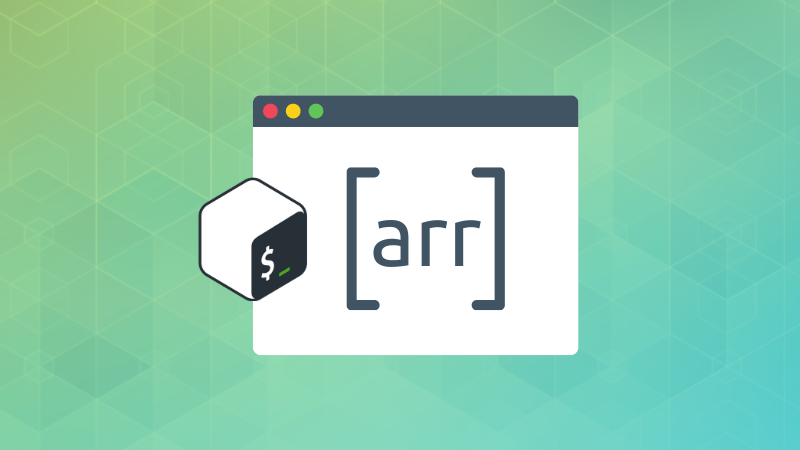
Method 2: Count elements using the for loop (not recommended)
Using a for loop, the number of elements in an array can be found. It is pretty simple, but don't use this method in general unless you're doing more than just counting.
The loop is as below:
#!/bin/bash
# Array
programs=("ip" "bat" "exa" "neofetch")
# Loop to count elements
len=0
for x in ${programs[@]}; do
((len++))
done
# Print count
echo "array is $len elements long"
programs
is an example arraylen
is the variable used to count elements (initialising with the value 0 so that it can count)for .... done
is the loop to go through the array and count each element. It increments the variablelen
for each element.- Then the length is printed in the end.
Remember when I mentioned don't use this method? Beucase it doesn't go well with huge data and causes problems in counting.
How loops take forever to count huge data
Learn more about Bash Scripting
Bash Basics is the series where everything you need to know about bash scripting is taught with interesting examples. If you're into Bash scripting, do check it out:
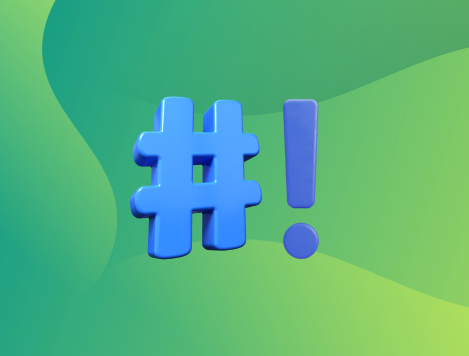
I hope you learned something from this article. Do drop your suggestions in the comments section.