In the earlier part of the series, you learned about variables. The variables can have a single value in it.
Arrays can have several values inside it. This makes things easier when you have to deal with several variables at a time. You don't have to store individual values in a new variable.
So, instead of declaring five variables like this:
distro1=Ubuntu
distro2=Fedora
distro3=SUSE
distro4=Arch Linux
distro5=Nix
You can initialize all of them in a single array:
distros=(Ubuntu Fedora SUSE "Arch Linux" Nix)
Unlike some other programming languages, you don't use commas as array element separators.
That's good. Let's see how to access the array elements.
Accessing array elements in bash
The array elements are accessed using the index (position in the array). To access array element at index N, use:
${array_name[N]}
nth
element has index n-1
.So, if you want to print the SUSE, you'll use:
echo ${distros[2]}
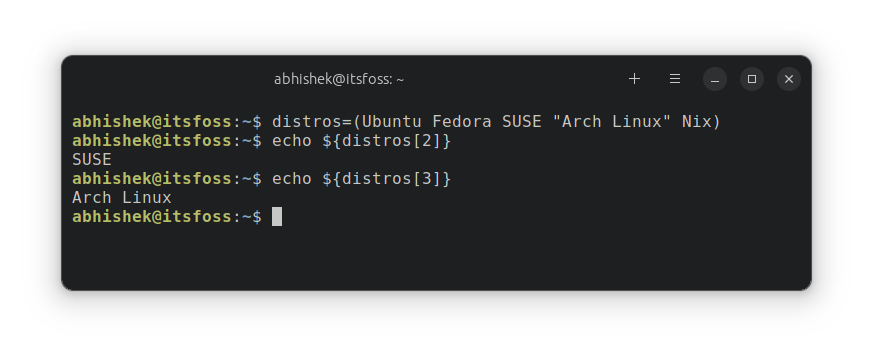
${
or before }
. You CANNOT use it like ${ array[n] }.Access all array elements at once
Let's say you want to print all the elements of an array.
You may use echo ${array[n]} one by one but that's really not necessary. There is a better and easier way:
${array[*]}
That will give you all the array elements.
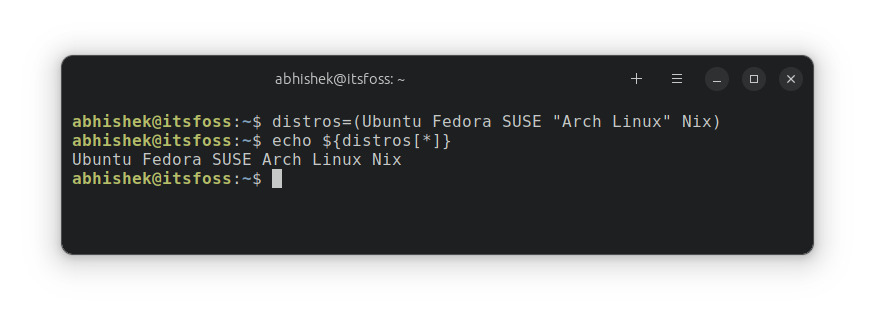
Get array length in bash
How do you know how many elements are there in an array? There is a dedicated way to get array length in Bash:
${#array_name[@]}
That's so simple, right?
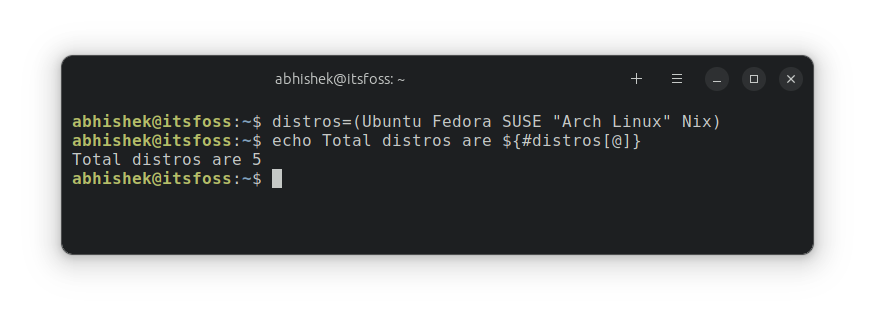
Add array elements in bash
If you have to add additional elements to an array, use the +=
operator to append element to existing array in bash:
array_name+=("new_value")
Here's an example:
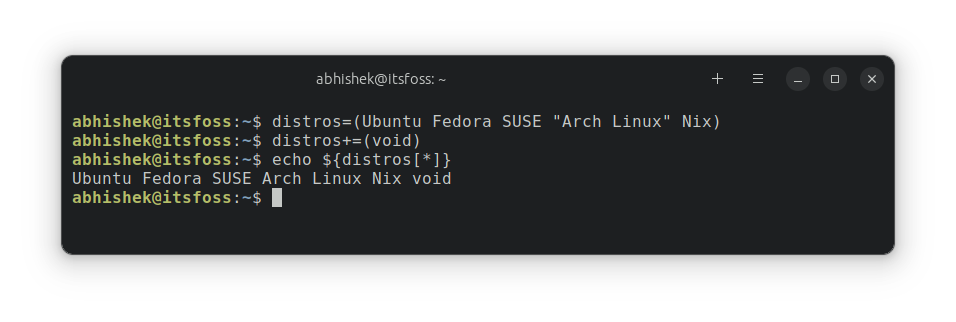
()
while appending an element.You can also use the index to set the element at any position.
array_name[N]=new_value
But remember to use the correct index number. If you use it on an existing index, the new value will replace the element.
If you use an 'out of bound' index, it will still be added after the last element. For example, if the array length is six and you try to set a new value at index 9, it will still be added as the last element at the 7th position (index 6).
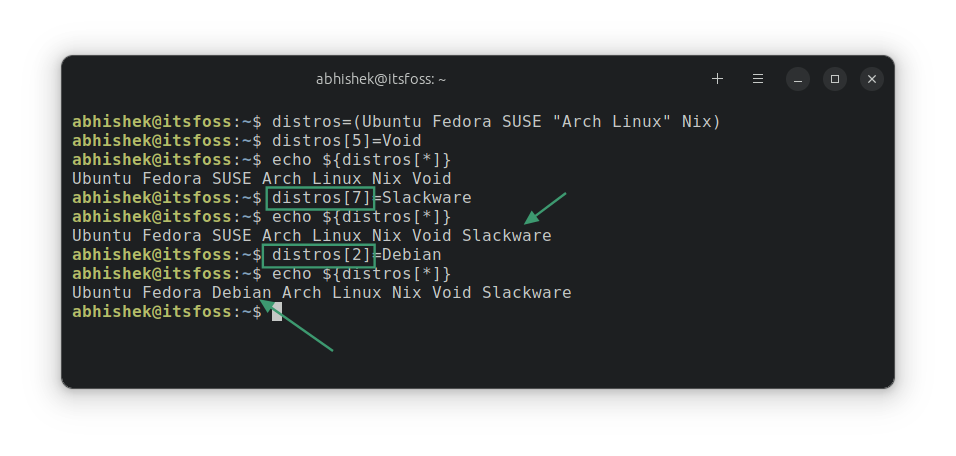
Delete an array element
You can use unset
shell built-in to remove an array element by providing the index number:
unset array_name[N]
Here's an example, where I delete the 4th element of the array.
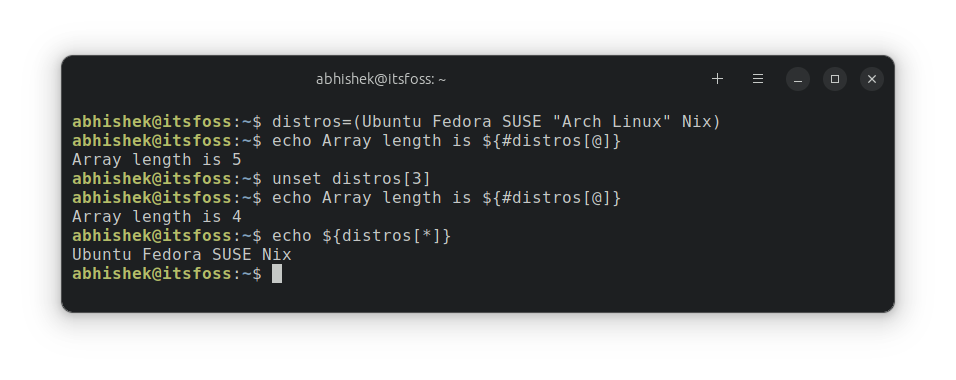
You can also delete the entire array with unset:
unset array_name
ποΈ Exercise time
Let's practice what you learned about bash arrays.
Exercise 1: Create a bash script that has an array of five best Linux distros. Print them all.
Now, replace the middle choice with Hannah Montanna Linux.
Exercise 2: Create a bash script that accepts three numbers from the user and then prints them in reverse order.
Expected output:
Enter three numbers and press enter
12 23 44
Numbers in reverse order are: 44 23 12
You can discuss your answers in the dedicated thread in the Community.
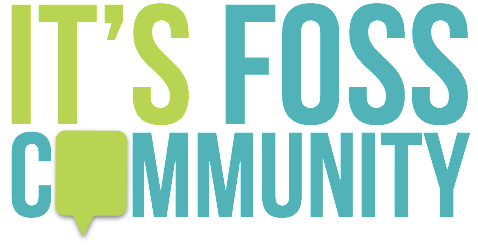
I hope you are enjoying learning bash shell scripting with this series. In the next chapter, you'll learn about handling strings in bash. Stay tuned.
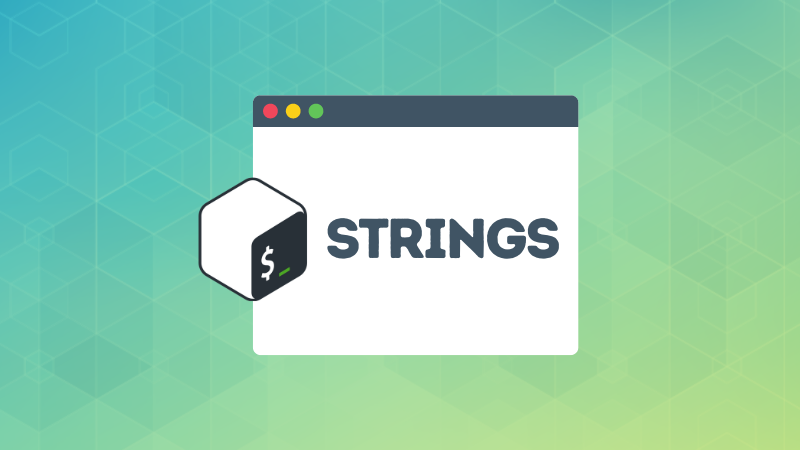