
When you think of strings in bash, you think of a word or a combination of a few words. Basically, a single line.
But what if you want to use a multiline strings in bash? I mean you have some text that goes over several lines.
Well, in this tutorial, I will walk you through two ways to create multiline strings in bash:
- Using heredocs
- Using printf
Let's start with the first one.
1. Using heredocs
Heredocs allow you to create multiline strings by using the cat command and a delimiter after the redirection operator (<<
). Sounds complex? Here's a simple syntax:
string_var=$(cat <<DELIMITER
This is a
multiline
string.
DELIMITER
)
echo "$string_var"
For example, here, I created a str
variable and used END
as a delimiter to create a multiline string:
str=$(cat <<END
Sagar Sharma
from
itsFOSS.com
END
)
echo "$str"
If you execute the above script, the output will look like this:
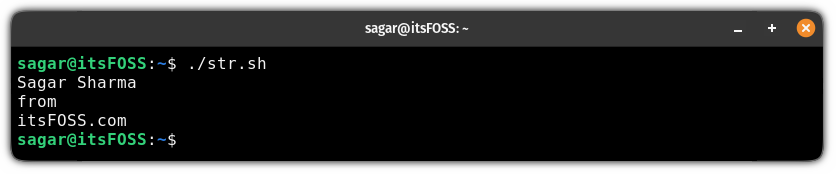
2. Using printf
With the printf command, you can print multiline strings by using a newline character (\n
) as shown here:
multiline_string="String-1\nString-2\nString-3."
Once after assigning multiline strings to a variable, you use the printf command with the -e
flag to enable the interpretation of backslash escape sequences.
multiline_string="String-1\nString-2\nString-3."
printf -e "$multiline_string"
For example, here, I created str_var
to hold 3 multiline strings and then execute the script to show its outcome:
str_var="Greetings\nFrom\nSagar Sharma."
echo -e "$str_var"
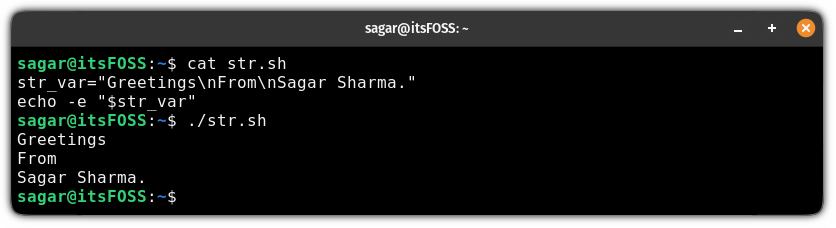
More on strings in bash
Ever wondered how you split a string in bash? Well, to help you with this, we wrote a detailed guide on how to split strings in bash:
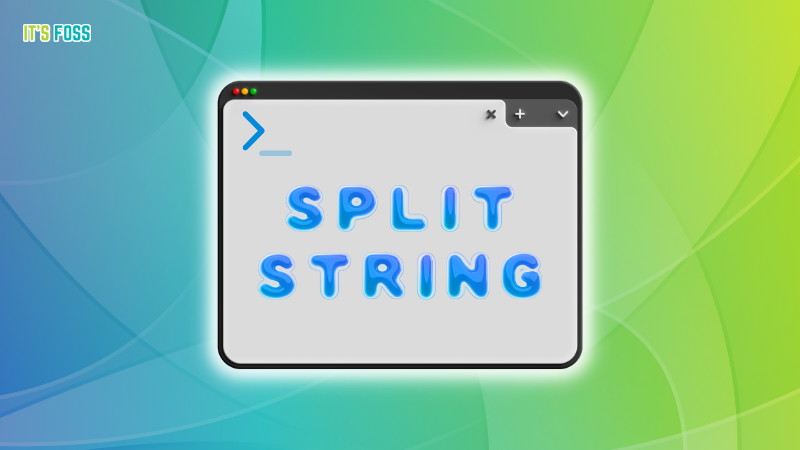
But if you are a beginner and want to know the basics of handling strings in bash, then refer to the following tutorial:
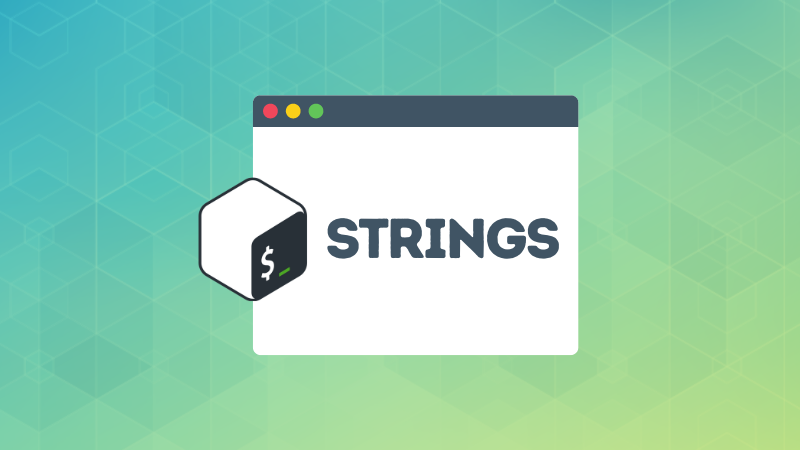
I hope you will find this guide helpful.