
You are here because you are writing a script in which you need to split a string, probably stored in a variable, and get at least two strings out of it.
Before I tell you how it works, know that the result will be an array that contains split strings. :)
With that, let's take a look at an example!
#!/usr/bin/env bash
my_string='Hello world'
IFS=' '
read -ra my_array <<< "${my_string}"
for substring in "${my_array[@]}"; do
echo "${substring}"
done
Assuming that the input string is Hello world
, upon executing this script, you will get the following output.
Hello
world
With that overview, let's take a look at what is happening.
Understanding how the string splitting worked
Let's take a look at a different but very similar script to understand what is happening.
#!/usr/bin/env bash
my_string='i,am,one,line,from,a,csv,file'
IFS=','
read -ra my_array <<< "${my_string}"
for substring in "${my_array[@]}"; do
echo "${substring}"
done
Here, I have taken the string i,am,one,line,from,a,csv,file
as the one I want to split.
The variable IFS
, is a special bash variable. It stands for Internal Field Separator. This variable holds what you would otherwise call a delimiter. Since I want to cause a split where a comma occurs, that is what I have assigned to the IFS
variable.
Now, about the actual splitting. That is done by the Bash built-in command: read
. Here, the -a
option is telling the read
command to store each word (that was split off) into a separate index in an array. The -r
option is optional but we use it to prevent escaping any characters that are preceded by a slash.
The my_array
is the array in which read
will store the words that are split. And doing a read <<< "${my_string}"
is the equivalent of doing echo "${my_string}" | read
. Since it is shorter, that is what I use.
Finally, I have a for
loop at the end that iterates over all indices of the array my_array
and prints it to the console one by one.
Conclusion
Bash allows you basic string manipulation. Splitting is not that straightforward, though.
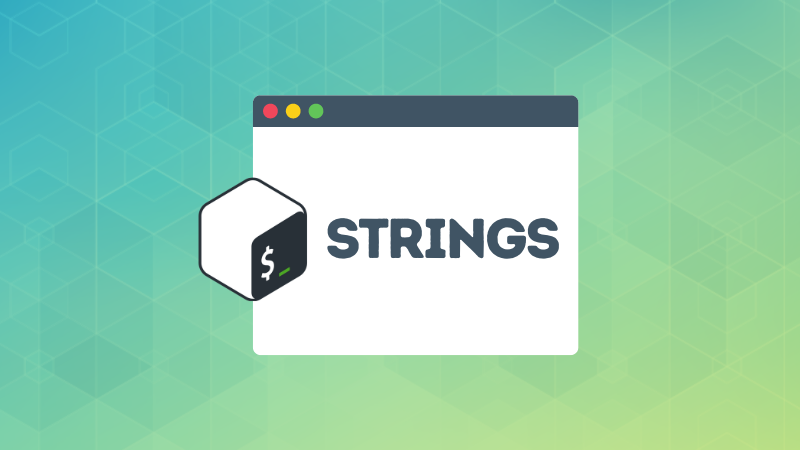
This concludes the method of string splitting in Bash using the read command. It is quite easy, but nonetheless, if you find any difficulties, please feel free to ask me in the comments! :)
It's FOSS turns 13! 13 years of helping people use Linux ❤️
And we need your help to go on for 13 more years. Support us with a Plus membership and enjoy an ad-free reading experience and get a Linux eBook for free.
To celebrate 13 years of It's FOSS, we have a lifetime membership option with reduced pricing of just $76. This is valid until 25th June only.
If you ever wanted to appreciate our work with Plus membership but didn't like the recurring subscription, this is your chance 😃