
Programming in Rust has taught me that checking if the variable is empty or not is always a good thing to do, before using the value (or absense of) inside.
To check if a variable is empty, use the -z
conditional operator. Following is an example demonstrating this:
if [[ -z "${MY_VAR}" ]]; then
echo "Empty variable, do not use it"
else
echo "Varible is not empty, safe to use it."
fi
You can use the -n
test operator to test if a variable is not empty.
To summarize:
Test condition | Description |
---|---|
-z | To check if var is empty |
-n | To check if var is not empty |
Let's see it in details with examples.
Checking if a variable is empty
Bash has a few conditional expressions, of which you can use one to check if a variable is empty or not. That is the -z
operator.
Using the -z
operator followed by a variable will result in true if the variable is empty. The condition will evaluate to false if the variable is not empty.
Let's take a look:
#!/usr/bin/env bash
EMPTY_VAR=''
NOT_EMPTY='1000101'
if [[ -z "${EMPTY_VAR}" ]]; then
echo "Variable 'EMPTY_VAR' is empty"
fi
if [[ ! -z "${NOT_EMPTY}" ]]; then
echo "Variable 'NOT_EMPTY' is populated"
fi
Here, I have two variables, one of which is empty and the other is populated. I am checking if the empty variable is empty and the non-empty variable is not empty :D
Upon running this script, I get the following output:
Variable 'EMPTY_VAR' is empty
Variable 'NOT_EMPTY' is populated
Checking if a variable is NOT empty
"What do you mean? Didn't you JUST cover it with ! -z
? I don't like redundant people!"
I get what you mean, but hear me out. What if I told you that there is a shorter way to check if a variable is not empty than what I demonstrated above. A ! -z
already exists: -n
.
The -n
option, accompanied with a varible, returns true if the variable is not empty.
Let's take a look at the same script, but with just one modification.
#!/usr/bin/env bash
EMPTY_VAR=''
NOT_EMPTY='1000101'
if [[ -z "${EMPTY_VAR}" ]]; then
echo "Variable 'EMPTY_VAR' is empty"
fi
if [[ -n "${NOT_EMPTY}" ]]; then
echo "Variable 'NOT_EMPTY' is populated"
fi
The only modification that I have made here is I replaced ! -z
with -n
. I expect the output to the be the same since the logic--pedantically speaking--is the same.
Here is what I see upon running the modified script:
Variable 'EMPTY_VAR' is empty
Variable 'NOT_EMPTY' is populated
New to Bash? Start here
If you are new to bash scripting, we have a good starting point for you in this Bash tutorial course that covers the essential concepts in the nine chapters.
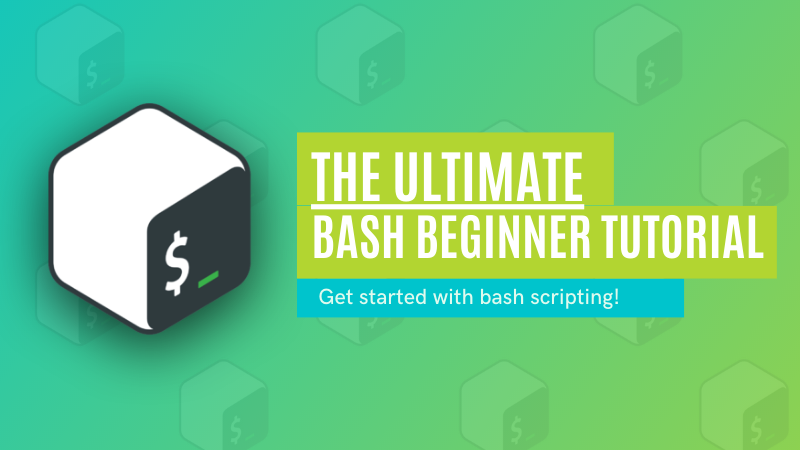
Enjoy bashing bash :)